Navigation
I have a player that I can move around and shoot using the keyboard, how does the enemy player I created do those same things. In Unity, its through the use of NavMes and NavMeshAgent. The NavMesh is a map of the walkable player surface on the level. The NavMeshAgent is the moving piece on the board. If an object has a NavMeshAgent component attched, it will automatically avoid other agents or obstacles it comes in contact with.
The navigation system needs to be aware of moving or stationary objects in order for the NavMeshAgent to alter the route. Using the NavMeshObstacle component to an object lets the system know that they need to be avoided.
More info on NavMeshObstacles: https://docs.unity3d.com/Manual/nav-CreateNavMeshObstacle.html
Creating a NavMesh is done by selecting the layer environment and changing it to Navigation Static in the Inspector window. Then you need to bake it. mmmmm smells good. Baking the NavMesh is done through the Windows -> AI -> Navigation menu. When I hit bake, a folder was created in the Scenes folder that contained all the lighting, navigation mesh, and reflection probe data for the scene. Now I got some funky lines showing up on the floor of the arena.
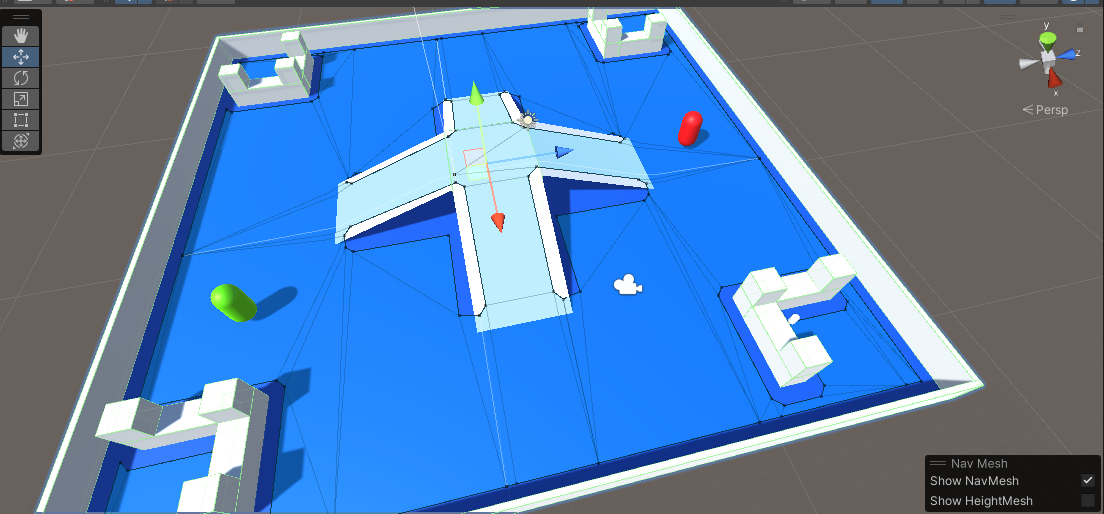
Lets get to making angry enemies. What kind of shooter would I have if I didn't have anyone shooting back.
Moving the Enemy
What the hell is procedural programming?? Apparently a common use for procedural programming is taking items from one collection to another modifying the item along the way. This is great for getting our bad guy to move between the four patrol points that we have in our game.
We're getting deep in the weeds of programming now. My head hurts. So after re-reading the section, it appears that we create a public Transform called PatrolRoute and a List transform called Locations. We next have a function that runs through a foreach loop on the child in PatrolRoute and add it to Locations. In Unity the Patrol_Route object is added to the Patrol Route variable in the EnemyBehavior section of the Enemy object.
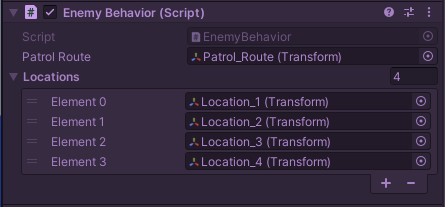
Before you start the game the Locations list above is empty. Once the play button is hit it takes the list of locations from the Patrol_Route object and populates the locations table as listed above. Using procedural programming makes the code safer and more readable.
Get a move on Soldier
Now that we have a list of places for the enemy to go, we have to figure out how to get him there. We're going to do this using the enemy's NavMeshAgent component. Ok so I made the guy move following the code in the book. I'm a bit confused right now. In the code I used a % operator which gives a remainder of two values being divided. So if 2 / 4 the remainder would be 2. This modulo operation is used to provide the next location for the enemy to travel to. The locations are indexed 0 - 4. So the enemy would start at 0 then increment by one each time as outlined in this section of the code.
void MoveToNextPatrolLocation()
{
if (Locations.Count == 0)
{
return;
}
_agent.destination = Locations[_locationIndex].position;
_locationIndex = (_locationIndex + 1) % Locations.Count;
}
Whew, that was a lot for today. At some point I would like to figure out how to randomize the location the enemy player is going to. Maybe that is a different section.